Add test case for enable toString object view (#593)
Signed-off-by: Jinbo Wang <jinbwan@microsoft.com>
This commit is contained in:
parent
ad82471da0
commit
58e11da115
|
@ -523,6 +523,13 @@ Exception in thread "main" java.lang.IllegalStateException
|
||||||
|
|
||||||
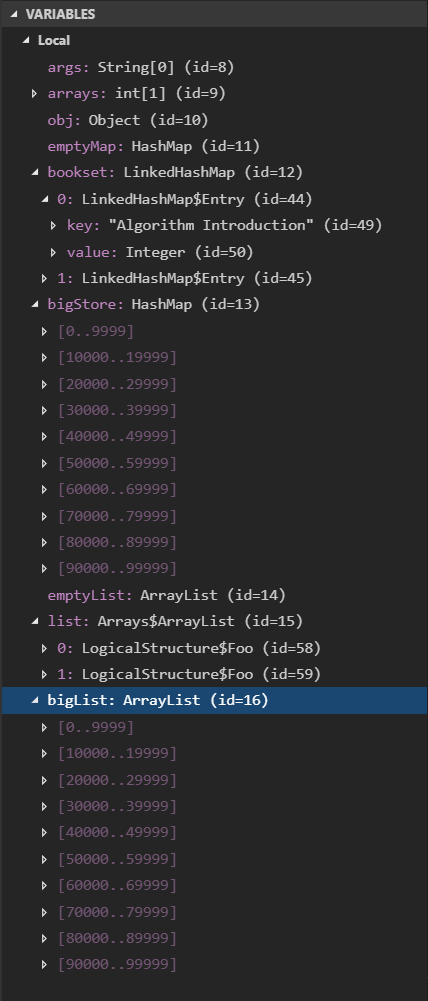
|
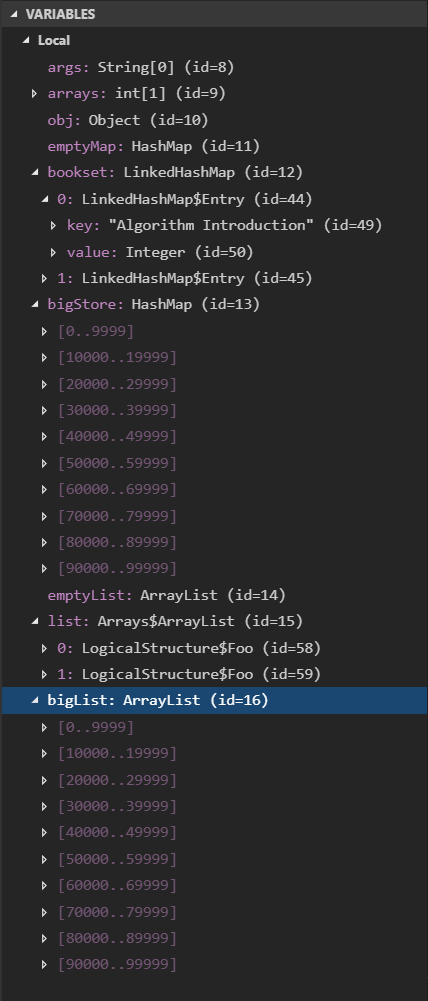
|
||||||
|
|
||||||
|
## Show toString object view
|
||||||
|
1. Open `28.debugfeatures` project in VS Code.
|
||||||
|
2. Open `Variables.java` file, and add a breakpoint at line 39.
|
||||||
|
3. Click Debug CodeLens, check the Variable viewlet.
|
||||||
|
4. Verify the highlight value in the screenshot below.
|
||||||
|
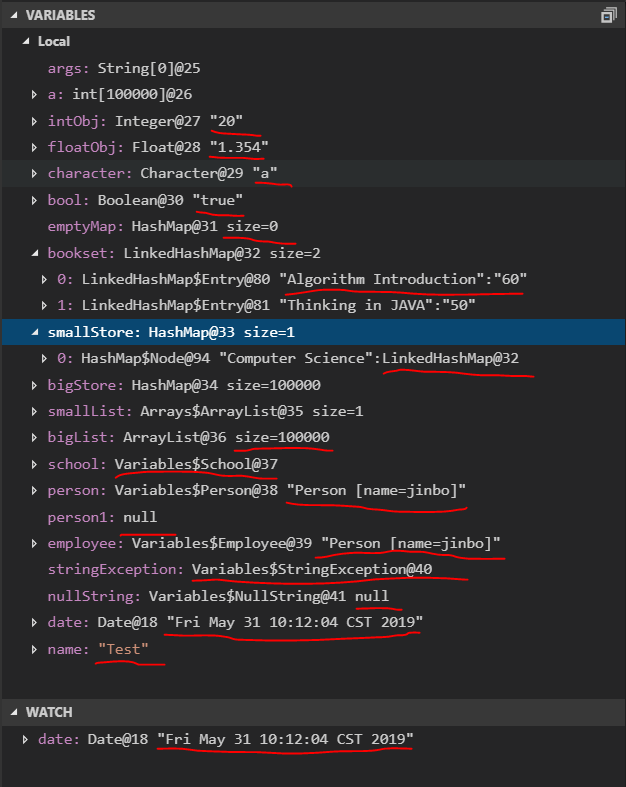
|
||||||
|
|
||||||
## Enable Java 12 preview for standalone Java files
|
## Enable Java 12 preview for standalone Java files
|
||||||
1. Install JDK-12.
|
1. Install JDK-12.
|
||||||
2. Open `28.debugfeatures` project in VS Code, and open `Java12Preview.java` file.
|
2. Open `28.debugfeatures` project in VS Code, and open `Java12Preview.java` file.
|
||||||
|
|
|
@ -0,0 +1,75 @@
|
||||||
|
import java.util.*;
|
||||||
|
|
||||||
|
public class Variables {
|
||||||
|
|
||||||
|
public static void main(String[] args) {
|
||||||
|
int[] a = new int[100000];
|
||||||
|
Integer intObj = new Integer(20);
|
||||||
|
Float floatObj = new Float("1.354");
|
||||||
|
Character character = new Character('a');
|
||||||
|
Boolean bool = new Boolean(true);
|
||||||
|
|
||||||
|
Map<String, String> emptyMap = new HashMap<>();
|
||||||
|
Map<String, Integer> bookset = new LinkedHashMap<>();
|
||||||
|
bookset.put("Algorithm Introduction", 60);
|
||||||
|
bookset.put("Thinking in JAVA", 50);
|
||||||
|
|
||||||
|
Map<String, Map<String, Integer>> smallStore = new HashMap<>();
|
||||||
|
smallStore.put("Computer Science", bookset);
|
||||||
|
|
||||||
|
Map<String, Map<String, Integer>> bigStore = new HashMap<>();
|
||||||
|
for (int i = 0; i < 100000; i++) {
|
||||||
|
bigStore.put("key" + i, bookset);
|
||||||
|
}
|
||||||
|
|
||||||
|
List<String> smallList = Arrays.asList("Algorithm Introduction");
|
||||||
|
List<String> bigList = new ArrayList<>();
|
||||||
|
for (int i = 0; i < 100000; i++) {
|
||||||
|
bigList.add("key" + i);
|
||||||
|
}
|
||||||
|
|
||||||
|
School school = new School();
|
||||||
|
Person person = new Person();
|
||||||
|
Person person1 = null;
|
||||||
|
Employee employee = new Employee();
|
||||||
|
StringException stringException = new StringException();
|
||||||
|
NullString nullString =new NullString();
|
||||||
|
Date date = new Date();
|
||||||
|
String name = "Test";
|
||||||
|
System.out.println("Exit.");
|
||||||
|
}
|
||||||
|
|
||||||
|
public static class School {
|
||||||
|
String name = "test";
|
||||||
|
|
||||||
|
}
|
||||||
|
|
||||||
|
public static class Person {
|
||||||
|
String name = "jinbo";
|
||||||
|
|
||||||
|
@Override
|
||||||
|
public String toString() {
|
||||||
|
return "Person [name=" + name + "]";
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
public static class Employee extends Person {
|
||||||
|
|
||||||
|
}
|
||||||
|
|
||||||
|
public static class StringException {
|
||||||
|
|
||||||
|
@Override
|
||||||
|
public String toString() {
|
||||||
|
throw new RuntimeException("Unimplemented method exception");
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
public static class NullString {
|
||||||
|
|
||||||
|
@Override
|
||||||
|
public String toString() {
|
||||||
|
return null;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
Loading…
Reference in New Issue