Remove test projects from main branch (#1046)
This commit is contained in:
parent
ee14dbe3d6
commit
a971831ea2
547
TestPlan.md
547
TestPlan.md
|
@ -1,547 +0,0 @@
|
|||
# Test Plan
|
||||
## Hello World
|
||||
|
||||
1. Create a new folder `helloworld`, create a file named `App.java` in this folder and filling the following codes:
|
||||
<pre>
|
||||
public class App
|
||||
{
|
||||
public static void main( String[] args )
|
||||
{
|
||||
System.out.println( "Hello World!" );
|
||||
}
|
||||
}
|
||||
</pre>
|
||||
|
||||
2. Set BP on println
|
||||
3. Press F5, verify for launch.json generated with right `mainClass`
|
||||
4. Press F5, verify for BP to be hit, verify variable args shows like `String[0] (id=%d)`
|
||||
5. Add watch `args.toString() + " test"`, verify the result like `[Ljava.lang.String;@726f3b58 test`
|
||||
6. Input `args.toString() + " test"` in debug console, verify the same result in debug console and it is expandable.
|
||||
7. Verify stack with thread list and on paused thread and one stack:
|
||||
`App.main(String[]) (App.java:5)`
|
||||
8. Press F10, verify `Hello World!` in debug console.
|
||||
9. Press F5, verify program terminates.
|
||||
|
||||
## Call Stack
|
||||
1. Open test project `2.callstack`
|
||||
2. Open `Bar.java`, set BP on line 3
|
||||
1. Press F5, verify for launch.json generated with right `projectName` and `mainClass`
|
||||
4. Press F5, verify for BP to be hit, verify variable args shows `i: 20` and `this: Bar (id=^d)`
|
||||
|
||||
1. Verify the stackframe shows:
|
||||
<pre>
|
||||
Bar.testBar(int) (Bar.java:3)
|
||||
Foo.testFoo(int) (Foo.java:6)
|
||||
CallStack.main(String[]) (CallStack.java:4)
|
||||
</pre>
|
||||
1. Click the testFoo line in the call stack view, verify vscode views verify variable args shows `j: 10` and `this: Foo (id=^d)` and `this` is expandable with only member `bar: Bar (id=^d)`, the bar should not be expandable.
|
||||
1. Change `j` to `32` and switch to stackframe `Bar.testBar(int) (Bar.java:3)` and switch back to `Foo.testFoo(int) (Foo.java:6)`, input `j + 1` in debug console and verify `33` is diplayed.
|
||||
1. Add watch `this.bar.toString()` and verify the result `"Bar@^d" (id=^d)`
|
||||
1. Add watch `"test" + new Bar().hashCode()` and verify the result `"test^d" (id=^d)`
|
||||
1. Add watch `bar.testBar(j + 10)` and verify there is a message `This is test method in bar.` printed in debug console.
|
||||
9. Press F5, verify program terminates.
|
||||
|
||||
## Call Stack
|
||||
1. Open test project `2.callstack`
|
||||
2. Open `Bar.java`, set BP on line 3
|
||||
1. Press F5, verify for launch.json generated with right `projectName` and `mainClass`
|
||||
4. Press F5, verify for BP to be hit, verify variable args shows `i: 20` and `this: Bar (id=^d)`
|
||||
|
||||
1. Verify the stackframe shows:
|
||||
<pre>
|
||||
Bar.testBar(int) (Bar.java:3)
|
||||
Foo.testFoo(int) (Foo.java:6)
|
||||
CallStack.main(String[]) (CallStack.java:4)
|
||||
</pre>
|
||||
1. Click the testFoo line in the call stack view, verify vscode views verify variable args shows `j: 10` and `this: Foo (id=^d)` and `this` is expandable with only member `bar: Bar (id=^d)`, the bar should not be expandable.
|
||||
1. Change `j` to `32` and switch to stackframe `Bar.testBar(int) (Bar.java:3)` and switch back to `Foo.testFoo(int) (Foo.java:6)`, input `j + 1` in debug console and verify `33` is diplayed.
|
||||
1. Add watch `this.bar.toString()` and verify the result `"Bar@^d" (id=^d)`
|
||||
1. Add watch `"test" + new Bar().hashCode()` and verify the result `"test^d" (id=^d)`
|
||||
1. Add watch `bar.testBar(j + 10)` and verify there is a message `This is test method in bar.` printed in debug console.
|
||||
9. Press F5, verify program terminates.
|
||||
|
||||
## Variables
|
||||
1. Open test project `4.variable`
|
||||
|
||||
1. Set BP on the last statement of `VariableTest#test`
|
||||
1. Press F5 to generate `launch.json` and press F5 again to start debug and wait for BP to be hit
|
||||
1. Verify
|
||||
1. `i` should be `111`
|
||||
1. `nullstr` should be `null`
|
||||
1. `str` should be full displayed with 1000 `a`
|
||||
1. `object` should be `Object (id=^d)` and not expandable
|
||||
1. `test` should be `VariableTest (id=^d)` and expandable with `x (VariableTest): 100`, `i:19099` and `x (Foo): 0`
|
||||
1. `a` is not expandable
|
||||
1. `b` is displayed as `Class (A) (id=^d)` and expand `b->classLoader`, there should be no errors on logs
|
||||
1. `intarray` should have 3 integers `1, 2, 3`
|
||||
1. `strList` should have children `size: 2` and expandable `elementData`, expand `elementData`, a long string `string test aaaaaaa...` should be displayed
|
||||
1. `map` should have `size: 1` and expandable `table` with a `HashMap$Node` child.
|
||||
1. `t` should have one element `hello`
|
||||
1. `genericArray` should be displayed `String[10][] (id=^d)` with first non-empty element
|
||||
1. `multi` should be displayed `String[5][][] (id=^d)` and two levels expandable tree nodes.
|
||||
1. `d` should not be expandable
|
||||
1. `dd` should be displayed as `GenericsFoo (id=^d)` and should have one child `x: Foo (id=^d)` with only one child `x: 0`
|
||||
1. `list` should have only one element of `int[1] (id=^d)` at index `0`
|
||||
1. `this` variable should be displayed
|
||||
1.
|
||||
1. set value on `test->x (VariableTest)`, input value `1`, verify `this->x (VariableTest)` has also been changed to `1`
|
||||
|
||||
1. Open user settings and set
|
||||
<pre>"java.debug.settings.showHex": true,
|
||||
"java.debug.settings.maxStringLength": 10,
|
||||
"java.debug.settings.showQualifiedNames": true,
|
||||
"java.debug.settings.showStaticVariables": false, </pre>
|
||||
1. verify
|
||||
1. numbers are displayed in hex, and static variable is hiden and class names have been changed to fully qualified, `str` should be changed to `"string ..." (id=0x^d)`
|
||||
2. add watch `this.hashCode()` verify the result should be hex format.
|
||||
1. add watch `str + str.length()` in debug console, verify the result `"string ..." (id=0x^d)`
|
||||
1. Press F5, verify program terminates.
|
||||
|
||||
## Big stack frame
|
||||
1. Open test project `6. recursivefunction`
|
||||
2. Open `RecursiveTest.java`, and set BP on line 8: return 1
|
||||
1. Press F5 to generate `launch.json` and press F5 again to start debug and wait for BP to be hit
|
||||
1. Add watch `number + 100` and verify the call stack list is a long list, and you can switch between them freely with changed arguments number and changed watch values
|
||||
1. click on the Load More Stack Frames button, verify you can click on it continually. (The total stack frame count is 1000, and vscode will load 20 of them in one page, so you can click Load More Stack Frames button about 50 times), verify there is no PERFORMANCE issue (no delay more than 1 second during the test steps).
|
||||
1. Press F5, verify program terminates.
|
||||
|
||||
## Breakpoint and innner class
|
||||
1. Open test project `5.breakpoint`
|
||||
1. Change the code to:
|
||||
```
|
||||
class A {
|
||||
|
||||
void m() {
|
||||
System.out.println("outer");
|
||||
}
|
||||
|
||||
String n() {
|
||||
return "outer";
|
||||
}
|
||||
}
|
||||
|
||||
public class BreakPointTest {
|
||||
public static void main(String[] args) {
|
||||
new BreakPointTest().go();
|
||||
int j = 0;
|
||||
new A() {
|
||||
@Override
|
||||
void m() {
|
||||
System.out.println("anonymous");
|
||||
}
|
||||
}.m();
|
||||
for (int i = 1; i <= 100; i++) {
|
||||
if (i <= 99) {
|
||||
j++;
|
||||
} else {
|
||||
System.out.println(j);
|
||||
}
|
||||
|
||||
}
|
||||
}
|
||||
|
||||
void go() {
|
||||
new A().m();
|
||||
class A {
|
||||
String n() {
|
||||
return "inner";
|
||||
}
|
||||
|
||||
void m() {
|
||||
System.out.println("inner");
|
||||
}
|
||||
}
|
||||
new A().m();
|
||||
}
|
||||
|
||||
static class A {
|
||||
String n() {
|
||||
return "middle";
|
||||
}
|
||||
|
||||
void m() {
|
||||
System.out.println("middle");
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
1. Set BP on each `println` statement
|
||||
1. Add watch `new A().n()`
|
||||
1. Press F5 to start debug, verify the line `System.out.println("middle");` is hit, verify the watch result is `middle`
|
||||
1. Press F5 again, verify the line `System.out.println("inner");` is hit, verify the watch result is displayed with red error message
|
||||
1. press F5 again and verify the line `System.out.println("anonymous");` is hit and the watch result is `middle`
|
||||
1. press F5 again and verify the line `System.out.println(j);` is hit and the watch result is `middle`
|
||||
1. Press F5, verify the program stops, verify no error in logs.
|
||||
1. verify the following messages in debug console <pre>
|
||||
middle
|
||||
inner
|
||||
anonymous
|
||||
99
|
||||
</pre>
|
||||
|
||||
## Variable Performance Test
|
||||
1. Open test project `7.variableperformance`
|
||||
2. Open `TooManyVariables.java`, and set BP on `System.out.println("variable perf test.")`
|
||||
1. Press F5 to generate `launch.json` and press F5 again to start debug
|
||||
1. Open debug view and expand the ‘this’ variable in variable view.
|
||||
1. Verify the time for expanding are less than 5 seconds
|
||||
1. Press F5, verify program terminates.
|
||||
|
||||
## No Debug Information Test
|
||||
1. Open test project `8.nosource`
|
||||
1. Open `NoSourceTest.java`, and set breakpoint on line 4: `System.out.println(i+10);`
|
||||
1. Press F5 to generate `launch.json` and press F5 again to start debug, verify the BP is hit
|
||||
1. Verify the following stack frames:
|
||||
<pre>
|
||||
NoSourceTest.lambda$0(Integer) (NoSourceTest.java:4)
|
||||
^d.accept(Object) (Unknown Source:-1)
|
||||
Foo.bar(int,int,Consumer) (Unknown Source:-1)
|
||||
NoSourceTest.main(String[]) (NoSourceTest.java:3)
|
||||
</pre>
|
||||
1. Select stack frame `Foo.bar(int,int,Consumer) (Unknown Source:-1)`, add watch `this.hashCode() - arg0` and verify an integer as the result, verify watch `new Foo()` results in `Foo (id=^d)`
|
||||
1. Press F5, verify program terminates.
|
||||
|
||||
## Maven Test
|
||||
1. Open cmd.exe and create an empty folder and run `mvn archetype:generate –DarchetypeArtifactId:maven-archetype-quickstart`
|
||||
1. On `Define value for property 'groupId'` please input `com.ms.samples`
|
||||
1. On `Define value for property 'artifactId'` please input `simple-app`
|
||||
1. For other options, press Enter
|
||||
1. Open generated `simple-app` folder using vscode
|
||||
1. Wait and verify `.project` and `.classpath` files and `target` folder are generated.
|
||||
1. Set BP on the `println`
|
||||
1. Press F5 to generate `launch.json` and press F5 again to start debug, verify the BP is hit
|
||||
1. Press F5, verify program terminates with output `Hello World!`
|
||||
|
||||
## Gradle Test
|
||||
1. create a new folder and create file `build.gradle` with the following text:
|
||||
<pre>
|
||||
apply plugin: 'java'
|
||||
|
||||
// Redefine where to look for app and test code
|
||||
// In this example, our code is structured as:
|
||||
// project
|
||||
// └── src
|
||||
// ├── main
|
||||
// │ ├── java
|
||||
// │ └── resources
|
||||
sourceSets {
|
||||
main.java.srcDirs = ['src/main/java']
|
||||
}
|
||||
</pre>
|
||||
1. copy the src/main folder from prevous test case `Maven Test` to this folder.
|
||||
1. Set BP on the `println`
|
||||
1. Press F5 to generate `launch.json` and press F5 again to start debug, verify the BP is hit
|
||||
1. Press F5, verify program terminates with output `Hello World!`
|
||||
|
||||
## PetClinic
|
||||
1. Clone code from `https://github.com/spring-projects/spring-petclinic.git`
|
||||
1. Open the cloned project and verify `.project` and `.classpath` files and `target` folder are generated.
|
||||
1. Set BP on `WelcomeController.java` on line `return "welcome";` and `main` on `PetClinicApplication.java`
|
||||
1. Press F5 to generate `launch.json` and press F5 again to start debug, verify the BP on `main` is hit.
|
||||
1. Press F5 and verify the BP on `main` is hit again.
|
||||
1. Wait for output `2017-11-23 20:23:25.230 INFO 9448 --- [ restartedMain] s.b.c.e.t.TomcatEmbeddedServletContainer : Tomcat started on port(s): 8080 (http)
|
||||
`
|
||||
1. Open IE and navigate to `http://localhost:8080`, verify the `welcome` method is hit
|
||||
1. Press F5 and verify page like `spring-petclinic/target/classes/templates/welcome.html` is displayed on IE with `bad` css.
|
||||
1. STOP debug and do the test again from step 4
|
||||
|
||||
## TODO application
|
||||
1. Clone code from `https://github.com/Microsoft/todo-app-java-on-azure.git`
|
||||
1. Open the cloned project and verify `.project` and `.classpath` files and `target` folder are generated.
|
||||
1. Open file `todo-app-java-on-azure\src\main\resources\application.properties` and replaces it with a test configuration(see my email attachment)
|
||||
1. Set BP on `TodoListController.java` on `addNewTodoItem(@RequestBody TodoItem item)` and `main` on `TodoApplication.java`
|
||||
1. Press F5 to generate `launch.json` and press F5 again to start debug, verify the BP on `main` is hit.
|
||||
1. Press F5 to continue, open IE and open `http://localhost:8080/#/TodoList` and add a todo, press Button `Add` and verify the BP on addNewTodoItem is hit, wait a little time to load the stack frame.
|
||||
1. Press F10 and then Press F5, verify the todo item is added.
|
||||
|
||||
|
||||
## Single file build
|
||||
1. Open file in folder `21.single-file`.(`Open folder` or `Open file`)
|
||||
2. Press F5, make sure it debugs well.
|
||||
3. update the src to introduce a compilation error. For example, change `String s = "1";` to `String s = 1;`. Then hit F5, check whether vscode pop out an error message "Build fails, do you want to proceed", click `abort`, make sure there is no more error message.
|
||||
|
||||
## Console application
|
||||
|
||||
1. Open project `23.console-app` in vscode.
|
||||
2. Press `F5` choose java and make sure `launch.json` is generated.
|
||||
3. Press `F5` again to start debug.
|
||||
4. See VSCode `DEBUG CONSOLE` view, verify the program is blocking at the line `Please input your name:`.
|
||||
5. Terminate debugger.
|
||||
6. Go to `launch.json`, change the option `console` to `integratedTerminal`.
|
||||
7. Press `F5` again.
|
||||
8. See VSCode `TERMINAL` view, and user can input his/her name there and the program continue to run.
|
||||
9. Terminate debugger.
|
||||
10.Go to `launch.json`, change the option `console` to `externalTerminal`.
|
||||
11. Press `F5` again, and the debugger will pop up an external terminal (e.g. cmd.exe).
|
||||
12. User can input his/her name there and the program continue to run.
|
||||
|
||||
## Java 9 modular application
|
||||
|
||||
1. In your PC, install latest java 9 JDK, configure `JAVA_HOME`.
|
||||
2. Open project `19.java9-app` in vscode.
|
||||
3. Press `F5` choose java and verify `launch.json` is auto generated.
|
||||
4. Press `F5` to start debug.
|
||||
5. Verify `breakpoint` and `step` work.
|
||||
6. Click `Call Stack`, it will open the associated source file in VSCode correctly.
|
||||
|
||||
## Multi-root
|
||||
|
||||
1. Clone code from https://github.com/spring-projects/spring-petclinic.git
|
||||
2. Clone code https://github.com/Microsoft/todo-app-java-on-azure.git
|
||||
3. Open both above projects in the same VSCode windows under workspace features
|
||||
4. Navigate to the Debug view
|
||||
5. Generate configuration for sprintclinic
|
||||
6. Generate configuration for TODO
|
||||
7. Check both launch.json to see the selected project's main are generated.
|
||||
|
||||
# Step Filters
|
||||
|
||||
1. Open project `19.java9-app` in vscode.
|
||||
2. Follow gif to verify step filters feature.
|
||||

|
||||
|
||||
The new gif:
|
||||

|
||||
|
||||
## Hot Code Replace
|
||||
- Manually trigger hot code replace
|
||||
1. Open project `24.hotCodeReplace` in vscode.
|
||||
2. Set breakpoints: NameProvider.java line 12; Person.java line 13.
|
||||
3. Press `F5` to start debug.
|
||||
4. The program stopped at the Person.java line 13.
|
||||
5. Change the value of the line "old" to "new", and save the document.
|
||||
6. Click the "Hot Code Replace" icon in the debug toolbar to trigger HCR. Check the breakpoint will stop at line 12 .
|
||||
7. Click F10 to step over, check the value of `res` on the debug view of local variable which should be `new`.
|
||||
|
||||
- Automatically trigger hot code replace
|
||||
1. Repeat step 1 ~ 4 above.
|
||||
2. Change `java.debug.settings.hotCodeReplace` to `auto`.
|
||||
3. Change the value of the line "old" to "new", and save the document.
|
||||
4. HCR will be automatically triggered. Check the breakpoint will stop at line 12 .
|
||||
5. Click F10 to step over, check the value of `res` on the debug view of local variable which should be `new`.
|
||||
|
||||
- Disable hot code replace
|
||||
1. Repeat step 1 ~ 4 above.
|
||||
2. Change `java.debug.settings.hotCodeReplace` to `never`.
|
||||
3. Change the value of the line "old" to "new", and save the document.
|
||||
4. Click F10 to step over, check the value of `res` on the debug view of local variable which should be `old`.
|
||||
|
||||
## Conditional Breakpoints
|
||||
|
||||
1. Open project `simple-java` in vscode, write the following code:
|
||||
<pre>
|
||||
package com.ms.samples;
|
||||
|
||||
/**
|
||||
* Hello world!
|
||||
*
|
||||
*/
|
||||
public class App
|
||||
{
|
||||
public static void main( String[] args )
|
||||
{
|
||||
int i = 0;
|
||||
for (; i <= 1000; i++) {
|
||||
if (i == 1000) {
|
||||
System.out.println( "Hello World!" );
|
||||
}
|
||||
}
|
||||
System.out.println( "Hello World!" );
|
||||
}
|
||||
}
|
||||
|
||||
</pre>
|
||||
|
||||
2. set conditional breakpoint on line 13 with condition `i ==1000`, F5 and wait the breakpoint to be hit
|
||||
|
||||

|
||||
|
||||
3. verify i equals 1000 in variable window.
|
||||
4. F5 and wait for program to exit.
|
||||
|
||||
## Restart Frame
|
||||
|
||||
1. Open project `25.restartFrame` in vscode.
|
||||
2. Set breakpoint: Person.java line 28
|
||||
3. Press `F5` to start debug.
|
||||
4. The program stopped at the Person.java line 28
|
||||
5. Open the debug view, find the call stack of current breakpoint
|
||||
6. Right click the `HelloWorld$1.run()`, choose `Restart Frame`. Result: It should fail with error message in the right corner.
|
||||
7. Right click the `Persona.getInternalName()`, choose `Restart Frame`. Result: The program stop at the entry of getInternalName
|
||||
7. Right click the `Persona.getName()`, choose `Restart Frame`. Result: The program stop at the entry of getName. The above call stacks are popped.
|
||||
|
||||
## Encoding Test for project under chinese directory
|
||||
|
||||
1. Find some project under chinese characters directory.
|
||||
2. Open it in vscode.
|
||||
3. Press `F5` to start debug.
|
||||
4. Verify the program can be launched normally.
|
||||
|
||||
|
||||
## Encoding Test for text file encoding
|
||||
|
||||
1. Open a hello world project, and print `System.out.println("中文字符3323")`.
|
||||
2. Press F5 to start debug.
|
||||
3. Verify the output in DEBUG CONSOLE view is correctly displayed.
|
||||
|
||||
|
||||
## Caught and Uncaught exceptions test
|
||||
|
||||
1. Open project `simple-java` in vscode, change code from line12 to line16 with following code:
|
||||
```
|
||||
for (; i <= 1000; i++) {
|
||||
if (i == 1) {
|
||||
throw new IllegalStateException();
|
||||
}
|
||||
}
|
||||
```
|
||||
2. Open debug view and tick both Uncaught exceptions and Caught exeptions
|
||||
3. Press F5, verify progress stop at line 14
|
||||
4. Press F5 again, verify following messages in debug console
|
||||
```
|
||||
Exception in thread "main" java.lang.IllegalStateException
|
||||
at com.ms.samples.App.main(App.java:14)
|
||||
```
|
||||
|
||||
|
||||
## Restart Frame
|
||||
|
||||
1. Open project `25.restartFrame` in vscode.
|
||||
2. Set breakpoint: Person.java line 28
|
||||
3. Press `F5` to start debug.
|
||||
4. The program stopped at the Person.java line 28
|
||||
5. Open the debug console, input `s` or `g` , the auto complete window will pop up with intellisense support.
|
||||
|
||||
## Logpoint
|
||||
|
||||
1. Open a project in vscode, and add a logpoint. The log message is the simple text message. If you want to print the expression, you need wrap it with a curly bracket `{ javaExpression }`.
|
||||
2. Launch java debugger and continue your program.
|
||||
3. When the logpoint code branch is hit, it just log the message to the console and doesn't stop your program.
|
||||
|
||||

|
||||
|
||||
|
||||
## Start without debugging
|
||||
1. Open a Hello world project in VS Code.
|
||||
2. Set a breakpoint.
|
||||
3. Press <kbd>Ctrl+F5</kbd> to start without debugging.
|
||||
4. Verify that the program starts without hitting the breakpoint.
|
||||
5. Open a spring-boot project in VS Code.
|
||||
6. Press <kbd>Ctrl+F5</kbd> to start without debugging.
|
||||
7. Verify that the program starts, and there is no information in "watch" and "callstack" view.
|
||||
8. Click the "pause" button in the toolbar, it should NOT pause the program.
|
||||
9. Click the "stop" button in the toolbar, it should stop the program.
|
||||
|
||||
|
||||
## Environment Variables
|
||||
1. Open `26.environmentVariables` in vscode.
|
||||
2. Press <kbd>F5</kbd> to start.
|
||||
3. Verify the output in Debug Console should be as following:
|
||||
```
|
||||
CustomEnv: This env is for test plan.
|
||||
SystemPath: <value of PATH >
|
||||
FileEnv: Successfully loaded an env from a file.
|
||||
```
|
||||
|
||||
## Runtime classpath entry
|
||||
1. Open `27.runtimeClassEntry` in vscode.
|
||||
2. Press <kbd>F5</kbd> to start.
|
||||
3. Verify the output in Debug Console should be as following:
|
||||
```
|
||||
Tomcat started on port(s): 8080 (http)
|
||||
```
|
||||
|
||||
|
||||
|
||||
## Resolve Variables
|
||||
1. Open `17.argstest` in vscode.
|
||||
2. Change the launch.json as following:
|
||||
```
|
||||
"mainClass": "test.${fileBasenameNoExtension}",
|
||||
"args": [ "\"${execPath}\"",
|
||||
"${env:APPDATA}",
|
||||
"${fileExtname}",
|
||||
"${workspaceRootFolderName}"]
|
||||
]
|
||||
```
|
||||
3. Make a BP at line one of main method, Keep ArgsTest.java open and press <kbd>F5</kbd> to start.
|
||||
3. Verify the `args` has the values:
|
||||
- "C:\Users\andxu\AppData\Local\Programs\Microsoft VS Code\Code.exe"
|
||||
- "C:\Users\andxu\AppData\Roaming"
|
||||
- ".json"
|
||||
- "17.argstest"
|
||||
|
||||
## Args and vmArgs
|
||||
1. Open `17.argstest` in vscode.
|
||||
2. Change the launch.json as following:
|
||||
```
|
||||
"mainClass": "test.ArgsTest",
|
||||
"args": ["a b", "foo \\\"bar"],
|
||||
"vmArgs": ["-Xms64M", "-Xmx128M", "-Dfoo= \\bar"]
|
||||
```
|
||||
3. Add the following statement to get the properties:
|
||||
```
|
||||
String foo = (String) System.getProperties().get("foo");
|
||||
```
|
||||
4. Press F5 to verify the variables should be like this:
|
||||
|
||||

|
||||
|
||||
## Classpath shortener for long classpath project
|
||||
1. Open `longclasspath` project in VS Code.
|
||||
2. Append the following config to the launch.json:
|
||||
```
|
||||
"shortenCommandLine": "none"
|
||||
```
|
||||
3. Click Run or Debug CodeLens.
|
||||
- On Windows, it should pop up an error box saying "CreateProcess error"=206, The filename or extension is too long".
|
||||
- On Linux and Macos, it may succeed.
|
||||
4. On Windows/Linux/Macos, modify the launch.json with the following combinations. Click Run/Debug both should succeed.
|
||||
```
|
||||
"shortenCommandLine": ""/"auto"/"jarmanifest"/"argfile", // argfile requires your Java version is 9 and higher.
|
||||
"console": ""/"internalConsole"/"integratedTerminal"/"externalTerminal"
|
||||
|
||||
```
|
||||
|
||||
## Jump to source code when clicking stack trace in DEBUG CONSOLE
|
||||
1. Open `28.debugfeatures` project in VS Code.
|
||||
2. Open `StackTrace.java` file.
|
||||
3. Click Run or Debug CodeLens, check the DEBUG CONSOLE. It's expected to render the source link for each stack trace line, and clicking the link should open the associated Java file in the editor.
|
||||
|
||||
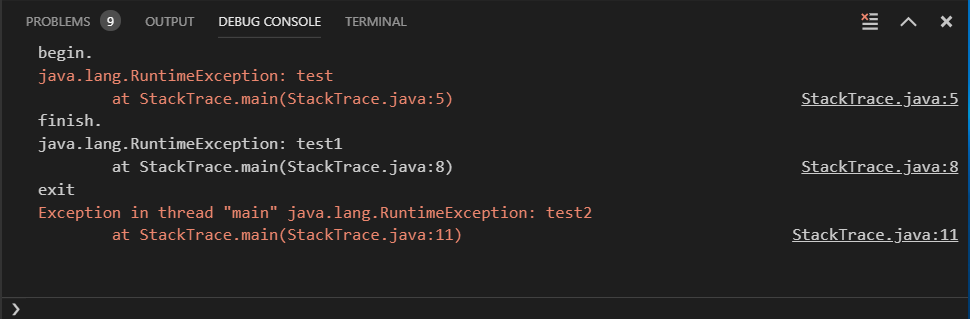
|
||||
|
||||
## Show the logical structure view for the Map and List variables
|
||||
1. Open `28.debugfeatures` project in VS Code.
|
||||
2. Open `LogicalStructure.java` file, and add a breakpoint at line 30.
|
||||
3. Click Debug CodeLens, check the Variable viewlet.
|
||||
- emptyMap - non-expandable
|
||||
- bookset - Show two children (0: LinkedHashMap$Entry, 1: LinkedHashMap$Entry)
|
||||
- bigStore - Lazy loading the children and show the index range first `[0..9999]` ...
|
||||
- emptyList - non-expandable
|
||||
- list - Show two children (0: LogicalStructure$Foo, 1: LogicalStructure$Foo).
|
||||
- bigList - Lazy loading the children and show the index range first `[0..9999]` ...
|
||||
|
||||
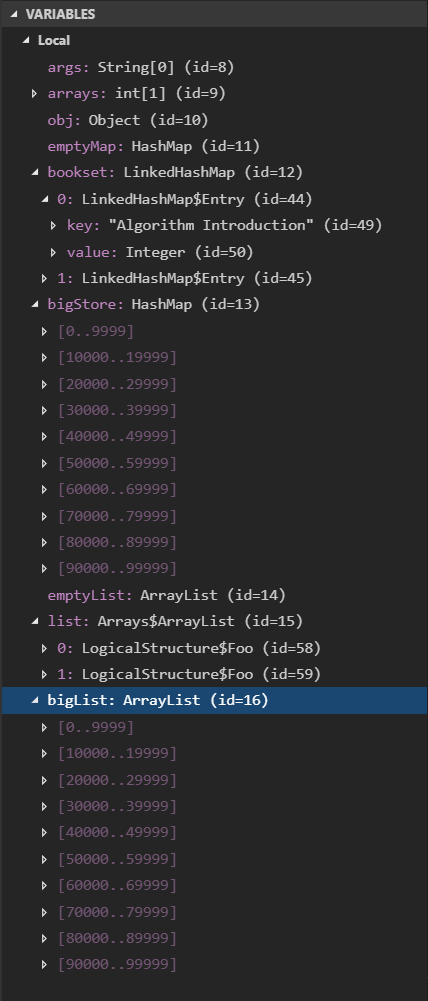
|
||||
|
||||
## Show toString object view
|
||||
1. Open `28.debugfeatures` project in VS Code.
|
||||
2. Open `Variables.java` file, and add a breakpoint at line 39.
|
||||
3. Click Debug CodeLens, check the Variable viewlet.
|
||||
4. Verify the highlight value in the screenshot below.
|
||||
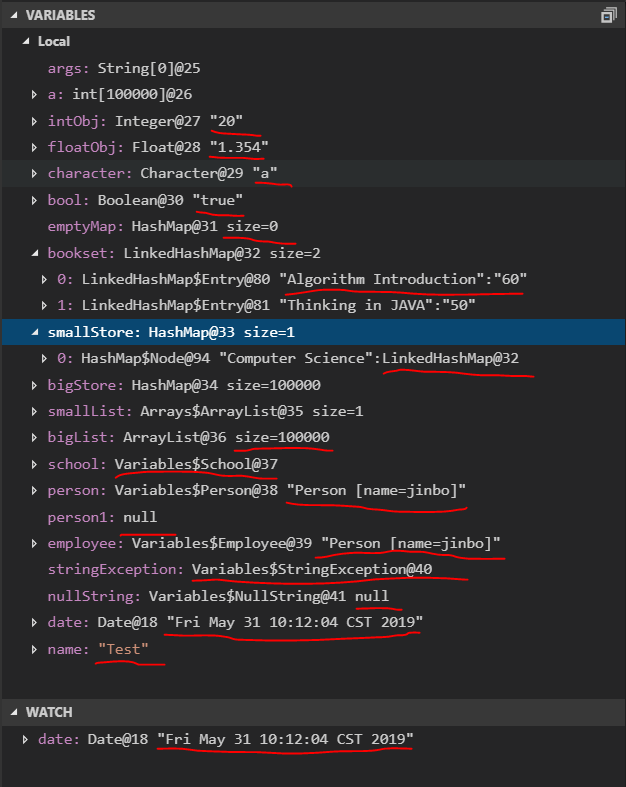
|
||||
|
||||
## Enable Java 12 preview for standalone Java files
|
||||
1. Install JDK-12.
|
||||
2. Open `28.debugfeatures` project in VS Code, and open `Java12Preview.java` file.
|
||||
3. Uncomment `"java.home"` in `./vscode/settings.json`.
|
||||
4. Run VS Code commmand `Java: clean Java language server workspace`, and click `Restart and delete` button in the prompted message box to reload VS Code.
|
||||
5. Add a breakpoint at line 7 of `Java12Preview.java`, and click Debug CodeLens. The debugger should run successfully.
|
||||
6. Open VS Code menu `Help -> Open Process Explorer`, find the Java Debuggger process in the `Process Explorer`. And its command line string should contain `--enable-preview` flag.
|
||||
|
||||
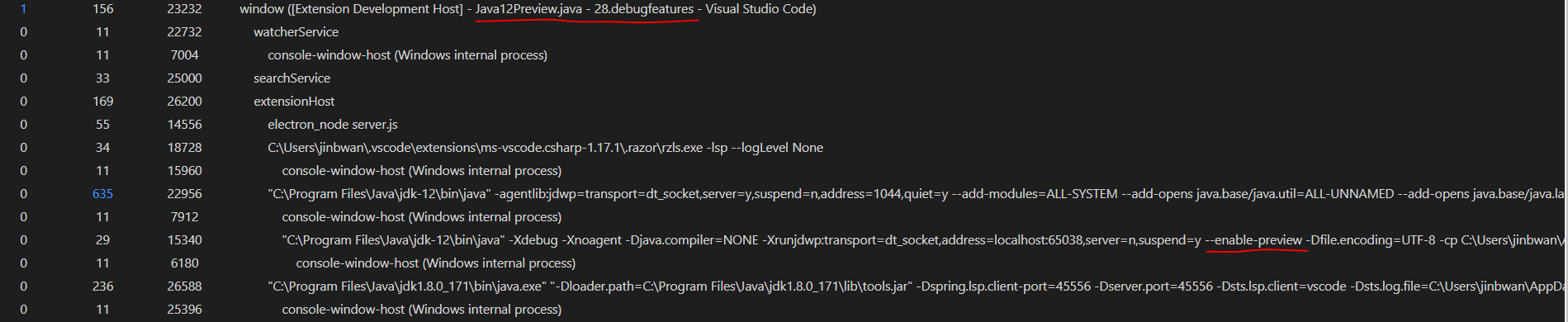
|
||||
|
||||
## Auto resolve classpath for maven projects
|
||||
1. Open `resolveClasspath` project in VS Code and wait for Java extensions are activated.
|
||||
2. Open `kie-client/src/main/java/client/EmbedMain.java` file, and click `Run` or `Debug` CodeLens to launch the application. Check DEBUG CONSOLE view to verify it is launched successfully.
|
||||
3. Open `insurance-decision/src/test/java/testscenario/Launch.java` file, and click `Run` or `Debug` CodeLens to launch the application. Check whether the message `Main Class in Test Folder!` is printed in DEBUG CONSOLE view.
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>1.helloworld</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,13 +0,0 @@
|
|||
public class HelloWorld {
|
||||
public static void main(String[] args) {
|
||||
System.out.print("hello");
|
||||
if (args != null && args.length > 0) {
|
||||
for (String arg : args) {
|
||||
System.out.print(" " + arg);
|
||||
}
|
||||
System.out.println();
|
||||
} else {
|
||||
System.out.println(" world");
|
||||
}
|
||||
}
|
||||
}
|
|
@ -1,9 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="src" path="src/test/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
<classpathentry sourcepath="lib/junit-4.12-sources.jar" kind="lib" path="lib/junit-4.12.jar"/>
|
||||
<classpathentry sourcepath="lib/hamcrest-core-1.3-sources.jar" kind="lib" path="lib/hamcrest-core-1.3.jar"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>10.junit</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,39 +0,0 @@
|
|||
apply plugin: 'java'
|
||||
apply plugin: 'eclipse'
|
||||
apply plugin: 'idea'
|
||||
|
||||
buildscript {
|
||||
repositories {
|
||||
mavenCentral()
|
||||
}
|
||||
dependencies { classpath "commons-io:commons-io:2.5" }
|
||||
}
|
||||
|
||||
import org.apache.commons.io.FilenameUtils;
|
||||
|
||||
sourceSets {
|
||||
main.java.srcDirs = ['src/main/java']
|
||||
}
|
||||
dependencies {
|
||||
testCompile 'junit:junit:4.12'
|
||||
}
|
||||
|
||||
|
||||
repositories {
|
||||
mavenCentral()
|
||||
}
|
||||
|
||||
def getShortJar = { e -> FilenameUtils.getName(e) }
|
||||
eclipse.classpath.file {
|
||||
withXml{xml ->
|
||||
def node = xml.asNode()
|
||||
|
||||
node.classpathentry.each{
|
||||
if (it.@kind == 'lib') {
|
||||
it.@path = 'lib/' + getShortJar(it.@path);
|
||||
it.@sourcepath = 'lib/' + getShortJar(it.@sourcepath);
|
||||
}
|
||||
|
||||
}
|
||||
}
|
||||
}
|
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
|
@ -1,17 +0,0 @@
|
|||
/*******************************************************************************
|
||||
* Copyright (c) 2017 Microsoft Corporation and others. All rights reserved.
|
||||
* This program and the accompanying materials are made available under the
|
||||
* terms of the Eclipse Public License v1.0 which accompanies this distribution,
|
||||
* and is available at http://www.eclipse.org/legal/epl-v10.html
|
||||
*
|
||||
* Contributors: Microsoft Corporation - initial API and implementation
|
||||
*******************************************************************************/
|
||||
|
||||
public class MyClass {
|
||||
public int multiply(int x, int y) {
|
||||
if (x > 999) {
|
||||
throw new IllegalArgumentException("X should be less than 1000");
|
||||
}
|
||||
return x * y;
|
||||
}
|
||||
}
|
|
@ -1,18 +0,0 @@
|
|||
import static org.junit.Assert.assertEquals;
|
||||
|
||||
import org.junit.Test;
|
||||
|
||||
public class MyTest {
|
||||
|
||||
@Test(expected = IllegalArgumentException.class)
|
||||
public void testExceptionIsThrown() {
|
||||
MyClass tester = new MyClass();
|
||||
tester.multiply(1000, 5);
|
||||
}
|
||||
|
||||
@Test
|
||||
public void testMultiply() {
|
||||
MyClass tester = new MyClass();
|
||||
assertEquals("10 x 5 must be 50", 50, tester.multiply(10, 5));
|
||||
}
|
||||
}
|
|
@ -1,18 +0,0 @@
|
|||
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
|
||||
<modelVersion>4.0.0</modelVersion>
|
||||
<groupId>com.mycompany.app</groupId>
|
||||
<artifactId>my-app</artifactId>
|
||||
<packaging>jar</packaging>
|
||||
<version>1.0-SNAPSHOT</version>
|
||||
<name>my-app</name>
|
||||
<url>http://maven.apache.org</url>
|
||||
<dependencies>
|
||||
<dependency>
|
||||
<groupId>junit</groupId>
|
||||
<artifactId>junit</artifactId>
|
||||
<version>3.8.1</version>
|
||||
<scope>test</scope>
|
||||
</dependency>
|
||||
</dependencies>
|
||||
</project>
|
|
@ -1,13 +0,0 @@
|
|||
package com.mycompany.app;
|
||||
|
||||
/**
|
||||
* Hello world!
|
||||
*
|
||||
*/
|
||||
public class App
|
||||
{
|
||||
public static void main( String[] args )
|
||||
{
|
||||
System.out.println( "Hello World!" );
|
||||
}
|
||||
}
|
|
@ -1,38 +0,0 @@
|
|||
package com.mycompany.app;
|
||||
|
||||
import junit.framework.Test;
|
||||
import junit.framework.TestCase;
|
||||
import junit.framework.TestSuite;
|
||||
|
||||
/**
|
||||
* Unit test for simple App.
|
||||
*/
|
||||
public class AppTest
|
||||
extends TestCase
|
||||
{
|
||||
/**
|
||||
* Create the test case
|
||||
*
|
||||
* @param testName name of the test case
|
||||
*/
|
||||
public AppTest( String testName )
|
||||
{
|
||||
super( testName );
|
||||
}
|
||||
|
||||
/**
|
||||
* @return the suite of tests being tested
|
||||
*/
|
||||
public static Test suite()
|
||||
{
|
||||
return new TestSuite( AppTest.class );
|
||||
}
|
||||
|
||||
/**
|
||||
* Rigourous Test :-)
|
||||
*/
|
||||
public void testApp()
|
||||
{
|
||||
assertTrue( true );
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
# Language server somehow recognizes build.gradle and compile this project.
|
||||
# Ignore following generated files to keep SCM clean.
|
||||
.gradle/
|
||||
.settings/
|
||||
.project
|
||||
.classpath
|
|
@ -1,6 +0,0 @@
|
|||
apply plugin: 'java'
|
||||
|
||||
|
||||
sourceSets {
|
||||
main.java.srcDirs = ['src/main/java']
|
||||
}
|
|
@ -1,5 +0,0 @@
|
|||
public class GradleTest {
|
||||
public static void main(String[] args) {
|
||||
System.out.println("This is a sample gradle project");
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry path="bin" kind="output"/>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>13.customcl</name>
|
||||
<comment></comment>
|
||||
<projects/>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments/>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<linkedResources/>
|
||||
<filteredResources/>
|
||||
</projectDescription>
|
Binary file not shown.
|
@ -1,56 +0,0 @@
|
|||
import java.io.ByteArrayOutputStream;
|
||||
import java.io.File;
|
||||
import java.io.IOException;
|
||||
import java.io.InputStream;
|
||||
import java.nio.file.Files;
|
||||
import java.nio.file.Paths;
|
||||
|
||||
class CustomCL extends ClassLoader {
|
||||
private String baseFolder;
|
||||
|
||||
public CustomCL(String baseFolder) {
|
||||
super(null);
|
||||
this.baseFolder = baseFolder;
|
||||
}
|
||||
|
||||
@Override
|
||||
protected Class<?> findClass(String name) throws ClassNotFoundException {
|
||||
// load from parent
|
||||
Class<?> result = findLoadedClass(name);
|
||||
if (result != null) {
|
||||
return result;
|
||||
}
|
||||
|
||||
try {
|
||||
File classFile = new File(baseFolder, name + ".class");
|
||||
if (classFile.exists()) {
|
||||
byte[] bytes = Files.readAllBytes(Paths.get(classFile.getAbsolutePath()));
|
||||
return defineClass(name, bytes, 0, bytes.length);
|
||||
}
|
||||
} catch (IOException e) {
|
||||
e.printStackTrace();
|
||||
}
|
||||
return getSystemClassLoader().loadClass(name);
|
||||
|
||||
}
|
||||
|
||||
@Override
|
||||
protected Class loadClass(String name, boolean resolve)
|
||||
throws ClassNotFoundException {
|
||||
Class cls;
|
||||
|
||||
cls = findLoadedClass(name);
|
||||
if (cls == null) {
|
||||
cls = findClass(name);
|
||||
}
|
||||
|
||||
if (cls == null) {
|
||||
throw new ClassNotFoundException(name);
|
||||
}
|
||||
|
||||
if (resolve)
|
||||
resolveClass(cls);
|
||||
return cls;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,26 +0,0 @@
|
|||
import java.io.File;
|
||||
|
||||
public class CustomClassLoaderTest {
|
||||
public static void main(String[] args) {
|
||||
File replacement = new File(new File("").getAbsolutePath(), "replacement");
|
||||
if (!replacement.exists()) {
|
||||
replacement = new File(new File("").getAbsolutePath(), "../replacement");
|
||||
}
|
||||
|
||||
|
||||
final String replacementFolder = replacement.getAbsolutePath();
|
||||
new Thread(()-> {
|
||||
try {
|
||||
System.out.println("Using " + replacementFolder);
|
||||
CustomCL cl = new CustomCL(replacementFolder);
|
||||
Class cls = cl.loadClass("Foo");
|
||||
|
||||
IFoo foo = (IFoo)cls.newInstance();
|
||||
new Foo().sayHello();
|
||||
foo.sayHello();
|
||||
} catch(Exception ex) {
|
||||
ex.printStackTrace();
|
||||
}
|
||||
}).start();
|
||||
}
|
||||
}
|
|
@ -1,5 +0,0 @@
|
|||
public class Foo implements IFoo {
|
||||
public void sayHello() {
|
||||
System.out.println("hello world! (version one)");
|
||||
}
|
||||
}
|
|
@ -1,3 +0,0 @@
|
|||
public interface IFoo {
|
||||
void sayHello();
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry path="bin" kind="output"/>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>14.encoding</name>
|
||||
<comment></comment>
|
||||
<projects/>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments/>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<linkedResources/>
|
||||
<filteredResources/>
|
||||
</projectDescription>
|
|
@ -1,2 +0,0 @@
|
|||
eclipse.preferences.version=1
|
||||
encoding//src/main/java/EncodingTest.java=gbk
|
|
@ -1,25 +0,0 @@
|
|||
import java.io.ByteArrayOutputStream;
|
||||
import java.io.IOException;
|
||||
import java.io.OutputStreamWriter;
|
||||
import java.nio.charset.Charset;
|
||||
|
||||
public class EncodingTest {
|
||||
public static void main(String[] args) throws Exception {
|
||||
String var = "abcÖÐÎÄdef";
|
||||
System.out.println(var);
|
||||
System.out.println(var.length());
|
||||
System.out.println("Default Charset=" + Charset.defaultCharset());
|
||||
System.out.println("file.encoding=" + System.getProperty("file.encoding"));
|
||||
System.out.println("Default Charset in Use=" + getDefaultCharSet());
|
||||
}
|
||||
|
||||
private static String getDefaultCharSet() {
|
||||
try (OutputStreamWriter writer = new OutputStreamWriter(new ByteArrayOutputStream())) {
|
||||
return writer.getEncoding();
|
||||
} catch (IOException ex) {
|
||||
ex.printStackTrace();
|
||||
return "exception";
|
||||
}
|
||||
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry path="bin" kind="output"/>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>15.specialname</name>
|
||||
<comment></comment>
|
||||
<projects/>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments/>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<linkedResources/>
|
||||
<filteredResources/>
|
||||
</projectDescription>
|
|
@ -1,6 +0,0 @@
|
|||
package ab$c;
|
||||
public class Hello$orld {
|
||||
public static void main(String args[]) {
|
||||
System.out.println("Hello$orld");
|
||||
}
|
||||
}
|
|
@ -1,8 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="src" path="src/test/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
<classpathentry sourcepath="lib/hamcrest-core-1.3-sources.jar" kind="lib" path="lib/hamcrest-core-1.3.jar"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>16.resolvemainclass</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,39 +0,0 @@
|
|||
apply plugin: 'java'
|
||||
apply plugin: 'eclipse'
|
||||
apply plugin: 'idea'
|
||||
|
||||
buildscript {
|
||||
repositories {
|
||||
mavenCentral()
|
||||
}
|
||||
dependencies { classpath "commons-io:commons-io:2.5" }
|
||||
}
|
||||
|
||||
import org.apache.commons.io.FilenameUtils;
|
||||
|
||||
sourceSets {
|
||||
main.java.srcDirs = ['src/main/java']
|
||||
}
|
||||
dependencies {
|
||||
testCompile 'junit:junit:4.12'
|
||||
}
|
||||
|
||||
|
||||
repositories {
|
||||
mavenCentral()
|
||||
}
|
||||
|
||||
def getShortJar = { e -> FilenameUtils.getName(e) }
|
||||
eclipse.classpath.file {
|
||||
withXml{xml ->
|
||||
def node = xml.asNode()
|
||||
|
||||
node.classpathentry.each{
|
||||
if (it.@kind == 'lib') {
|
||||
it.@path = 'lib/' + getShortJar(it.@path);
|
||||
it.@sourcepath = 'lib/' + getShortJar(it.@sourcepath);
|
||||
}
|
||||
|
||||
}
|
||||
}
|
||||
}
|
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
|
@ -1,14 +0,0 @@
|
|||
/*******************************************************************************
|
||||
* Copyright (c) 2017 Microsoft Corporation and others. All rights reserved.
|
||||
* This program and the accompanying materials are made available under the
|
||||
* terms of the Eclipse Public License v1.0 which accompanies this distribution,
|
||||
* and is available at http://www.eclipse.org/legal/epl-v10.html
|
||||
*
|
||||
* Contributors: Microsoft Corporation - initial API and implementation
|
||||
*******************************************************************************/
|
||||
|
||||
public class MyApp {
|
||||
public static void main(String agrsp[]) {
|
||||
System.out.println("Hello App!");
|
||||
}
|
||||
}
|
|
@ -1,7 +0,0 @@
|
|||
|
||||
public class MyAppTest {
|
||||
|
||||
public static void main(String agrsp[]) {
|
||||
System.out.println("Hello test App!");
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8"/>
|
||||
<classpathentry kind="src" path="src"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>17.argstest</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,11 +0,0 @@
|
|||
eclipse.preferences.version=1
|
||||
org.eclipse.jdt.core.compiler.codegen.inlineJsrBytecode=enabled
|
||||
org.eclipse.jdt.core.compiler.codegen.targetPlatform=1.8
|
||||
org.eclipse.jdt.core.compiler.codegen.unusedLocal=preserve
|
||||
org.eclipse.jdt.core.compiler.compliance=1.8
|
||||
org.eclipse.jdt.core.compiler.debug.lineNumber=generate
|
||||
org.eclipse.jdt.core.compiler.debug.localVariable=generate
|
||||
org.eclipse.jdt.core.compiler.debug.sourceFile=generate
|
||||
org.eclipse.jdt.core.compiler.problem.assertIdentifier=error
|
||||
org.eclipse.jdt.core.compiler.problem.enumIdentifier=error
|
||||
org.eclipse.jdt.core.compiler.source=1.8
|
|
@ -1,23 +0,0 @@
|
|||
package test;
|
||||
|
||||
import java.io.IOException;
|
||||
import java.util.ArrayList;
|
||||
import java.util.Arrays;
|
||||
import java.util.List;
|
||||
|
||||
public class ArgsTest {
|
||||
|
||||
public static void main(String[] args) throws IOException {
|
||||
|
||||
List<String> proList=Arrays.asList(args);
|
||||
|
||||
String sysProp1Value = System.getProperty("sysProp1");
|
||||
String sysProp2Value = System.getProperty("sysProp2");
|
||||
List<String> vmList=new ArrayList<>();
|
||||
vmList.add(sysProp1Value );
|
||||
vmList.add(sysProp2Value );
|
||||
String encoding=System.getProperty("file.encoding");
|
||||
System.out.println("Program Arguments:"+String.join(" ", proList)+" VM Arguments:"+String.join(" ",vmList));
|
||||
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8"/>
|
||||
<classpathentry kind="src" path="src"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>18.attachdebug</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,11 +0,0 @@
|
|||
eclipse.preferences.version=1
|
||||
org.eclipse.jdt.core.compiler.codegen.inlineJsrBytecode=enabled
|
||||
org.eclipse.jdt.core.compiler.codegen.targetPlatform=1.8
|
||||
org.eclipse.jdt.core.compiler.codegen.unusedLocal=preserve
|
||||
org.eclipse.jdt.core.compiler.compliance=1.8
|
||||
org.eclipse.jdt.core.compiler.debug.lineNumber=generate
|
||||
org.eclipse.jdt.core.compiler.debug.localVariable=generate
|
||||
org.eclipse.jdt.core.compiler.debug.sourceFile=generate
|
||||
org.eclipse.jdt.core.compiler.problem.assertIdentifier=error
|
||||
org.eclipse.jdt.core.compiler.problem.enumIdentifier=error
|
||||
org.eclipse.jdt.core.compiler.source=1.8
|
|
@ -1,14 +0,0 @@
|
|||
package test;
|
||||
import java.util.stream.Stream;
|
||||
|
||||
public class attachdebug {
|
||||
public static void main(String[] args) {
|
||||
String concat = Stream.of("A", "B", "C", "D").reduce("", String::concat);
|
||||
Integer[] sixNums = {1, 2, 3, 4, 5, 6};
|
||||
int evens =
|
||||
Stream.of(sixNums).filter(n -> n%2 == 0).reduce(0, Integer::sum);
|
||||
|
||||
System.out.println(concat+" "+evens);
|
||||
}
|
||||
|
||||
}
|
|
@ -1,39 +0,0 @@
|
|||
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
|
||||
<modelVersion>4.0.0</modelVersion>
|
||||
<groupId>com.microsoft.app</groupId>
|
||||
<artifactId>java9-app</artifactId>
|
||||
<packaging>jar</packaging>
|
||||
<version>1.0-SNAPSHOT</version>
|
||||
<name>java9-app</name>
|
||||
<url>http://maven.apache.org</url>
|
||||
<dependencies>
|
||||
<dependency>
|
||||
<groupId>junit</groupId>
|
||||
<artifactId>junit</artifactId>
|
||||
<version>3.8.1</version>
|
||||
<scope>test</scope>
|
||||
</dependency>
|
||||
<dependency>
|
||||
<groupId>com.google.code.gson</groupId>
|
||||
<artifactId>gson</artifactId>
|
||||
<version>2.7</version>
|
||||
</dependency>
|
||||
</dependencies>
|
||||
|
||||
<build>
|
||||
<plugins>
|
||||
<plugin>
|
||||
<groupId>org.apache.maven.plugins</groupId>
|
||||
<artifactId>maven-compiler-plugin</artifactId>
|
||||
<version>3.7.0</version>
|
||||
<configuration>
|
||||
<source>9</source>
|
||||
<target>9</target>
|
||||
<showWarnings>true</showWarnings>
|
||||
<showDeprecation>true</showDeprecation>
|
||||
</configuration>
|
||||
</plugin>
|
||||
</plugins>
|
||||
</build>
|
||||
</project>
|
|
@ -1,22 +0,0 @@
|
|||
package com.microsoft.app;
|
||||
|
||||
import com.google.gson.Gson;
|
||||
|
||||
public class App
|
||||
{
|
||||
public static void main( String[] args )
|
||||
{
|
||||
Gson GSON = new Gson();
|
||||
String name = "jinbwan";
|
||||
Employee employee = GSON.fromJson("{\"name\": \"" + name + "\"}", Employee.class);
|
||||
System.out.println(employee.getName());
|
||||
}
|
||||
|
||||
static class Employee {
|
||||
public String name;
|
||||
|
||||
public String getName() {
|
||||
return this.name;
|
||||
}
|
||||
}
|
||||
}
|
|
@ -1,11 +0,0 @@
|
|||
package com.microsoft.app;
|
||||
|
||||
import java.nio.file.Paths;
|
||||
|
||||
public class Launcher {
|
||||
public static void main(String[] args) {
|
||||
System.out.println(Paths.get("").toAbsolutePath().toString());
|
||||
|
||||
System.out.println(System.getenv("Path"));
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
module com.microsoft.app.mymodule {
|
||||
requires gson;
|
||||
requires java.base;
|
||||
requires java.sql;
|
||||
opens com.microsoft.app;
|
||||
}
|
|
@ -1,38 +0,0 @@
|
|||
package com.microsoft.app;
|
||||
|
||||
import junit.framework.Test;
|
||||
import junit.framework.TestCase;
|
||||
import junit.framework.TestSuite;
|
||||
|
||||
/**
|
||||
* Unit test for simple App.
|
||||
*/
|
||||
public class AppTest
|
||||
extends TestCase
|
||||
{
|
||||
/**
|
||||
* Create the test case
|
||||
*
|
||||
* @param testName name of the test case
|
||||
*/
|
||||
public AppTest( String testName )
|
||||
{
|
||||
super( testName );
|
||||
}
|
||||
|
||||
/**
|
||||
* @return the suite of tests being tested
|
||||
*/
|
||||
public static Test suite()
|
||||
{
|
||||
return new TestSuite( AppTest.class );
|
||||
}
|
||||
|
||||
/**
|
||||
* Rigourous Test :-)
|
||||
*/
|
||||
public void testApp()
|
||||
{
|
||||
assertTrue( true );
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry path="bin" kind="output"/>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>2.callstack</name>
|
||||
<comment></comment>
|
||||
<projects/>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments/>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<linkedResources/>
|
||||
<filteredResources/>
|
||||
</projectDescription>
|
|
@ -1,5 +0,0 @@
|
|||
public class Bar {
|
||||
public void testBar(int i) {
|
||||
System.out.println("This is test method in bar.");
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
public class CallStack {
|
||||
public static void main(String[] args) {
|
||||
Foo foo = new Foo();
|
||||
foo.testFoo(10);
|
||||
}
|
||||
}
|
|
@ -1,9 +0,0 @@
|
|||
public class Foo {
|
||||
private Bar bar = new Bar();
|
||||
|
||||
public void testFoo(int j) {
|
||||
System.out.println("This is test method in foo.");
|
||||
bar.testBar(j + 10);
|
||||
}
|
||||
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>20.usersettings</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,13 +0,0 @@
|
|||
package usersettings;
|
||||
|
||||
public class UserSettings {
|
||||
private static int number = 20;
|
||||
|
||||
public static void main(String[] args) {
|
||||
|
||||
String testName = "usersettings";
|
||||
System.out.println("Test name is " + testName + " and the test number is " + number);
|
||||
|
||||
}
|
||||
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>21.evaluate</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,14 +0,0 @@
|
|||
package evaluate;
|
||||
|
||||
public class EvaluateTest {
|
||||
public static void main(String[] args) {
|
||||
int i = 0;
|
||||
i++;
|
||||
int test1 = new EvaluateTest().test();
|
||||
System.out.print(test1 + i);
|
||||
}
|
||||
|
||||
public static int test() {
|
||||
return 3;
|
||||
}
|
||||
}
|
|
@ -1,14 +0,0 @@
|
|||
public class Main{
|
||||
public static void main(String[] args) {
|
||||
String s = "1";
|
||||
char sr = 'c';
|
||||
int t = 1;
|
||||
t++;
|
||||
test(t);
|
||||
}
|
||||
|
||||
private static void test(int t) {
|
||||
int s = 1;
|
||||
System.out.println("test" + s + t);
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>22.jdkversion</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,13 +0,0 @@
|
|||
import java.util.Arrays;
|
||||
import java.util.List;
|
||||
|
||||
public class JdkVersion {
|
||||
public static void main(String[] args) {
|
||||
String jdkVersion = System.getProperty("java.version");
|
||||
|
||||
List<Integer> nums = Arrays.asList(1, 2, 3, 4);
|
||||
float squareNums = nums.stream().map(n -> n * n).reduce((sum, n) -> sum + n).get().floatValue();
|
||||
System.out.println(jdkVersion);
|
||||
System.out.println(squareNums);
|
||||
}
|
||||
}
|
|
@ -1,18 +0,0 @@
|
|||
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
|
||||
<modelVersion>4.0.0</modelVersion>
|
||||
<groupId>com.microsoft.test</groupId>
|
||||
<artifactId>console-app</artifactId>
|
||||
<packaging>jar</packaging>
|
||||
<version>1.0-SNAPSHOT</version>
|
||||
<name>console-app</name>
|
||||
<url>http://maven.apache.org</url>
|
||||
<dependencies>
|
||||
<dependency>
|
||||
<groupId>junit</groupId>
|
||||
<artifactId>junit</artifactId>
|
||||
<version>3.8.1</version>
|
||||
<scope>test</scope>
|
||||
</dependency>
|
||||
</dependencies>
|
||||
</project>
|
|
@ -1,16 +0,0 @@
|
|||
package com.microsoft.test;
|
||||
|
||||
import java.util.Scanner;
|
||||
|
||||
public class App
|
||||
{
|
||||
public static void main( String[] args )
|
||||
{
|
||||
System.out.println("Please input your name:");
|
||||
|
||||
Scanner in = new Scanner(System.in);
|
||||
String name = in.nextLine();
|
||||
|
||||
System.out.println("Thanks, " + name);
|
||||
}
|
||||
}
|
|
@ -1,38 +0,0 @@
|
|||
package com.microsoft.test;
|
||||
|
||||
import junit.framework.Test;
|
||||
import junit.framework.TestCase;
|
||||
import junit.framework.TestSuite;
|
||||
|
||||
/**
|
||||
* Unit test for simple App.
|
||||
*/
|
||||
public class AppTest
|
||||
extends TestCase
|
||||
{
|
||||
/**
|
||||
* Create the test case
|
||||
*
|
||||
* @param testName name of the test case
|
||||
*/
|
||||
public AppTest( String testName )
|
||||
{
|
||||
super( testName );
|
||||
}
|
||||
|
||||
/**
|
||||
* @return the suite of tests being tested
|
||||
*/
|
||||
public static Test suite()
|
||||
{
|
||||
return new TestSuite( AppTest.class );
|
||||
}
|
||||
|
||||
/**
|
||||
* Rigourous Test :-)
|
||||
*/
|
||||
public void testApp()
|
||||
{
|
||||
assertTrue( true );
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>HelloWorld</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,11 +0,0 @@
|
|||
eclipse.preferences.version=1
|
||||
org.eclipse.jdt.core.compiler.codegen.inlineJsrBytecode=enabled
|
||||
org.eclipse.jdt.core.compiler.codegen.targetPlatform=1.8
|
||||
org.eclipse.jdt.core.compiler.codegen.unusedLocal=preserve
|
||||
org.eclipse.jdt.core.compiler.compliance=1.8
|
||||
org.eclipse.jdt.core.compiler.debug.lineNumber=generate
|
||||
org.eclipse.jdt.core.compiler.debug.localVariable=generate
|
||||
org.eclipse.jdt.core.compiler.debug.sourceFile=generate
|
||||
org.eclipse.jdt.core.compiler.problem.assertIdentifier=error
|
||||
org.eclipse.jdt.core.compiler.problem.enumIdentifier=error
|
||||
org.eclipse.jdt.core.compiler.source=1.8
|
|
@ -1,64 +0,0 @@
|
|||
package com.microsoft.debug;
|
||||
|
||||
|
||||
public class HelloWorld {
|
||||
static class InnerType {
|
||||
public static void print() {
|
||||
System.out.println("Inner Type.");
|
||||
}
|
||||
}
|
||||
|
||||
private static int value = 0;
|
||||
|
||||
public static void main(String[] args) throws Exception {
|
||||
new Thread() {
|
||||
public void run() {
|
||||
while (true) {
|
||||
try {
|
||||
Thread.sleep(500);
|
||||
|
||||
System.out.println("thread 1");
|
||||
|
||||
Person person = new Person("c4");
|
||||
System.out.println(person.getName());
|
||||
person.toString();
|
||||
} catch (InterruptedException e) {
|
||||
}
|
||||
}
|
||||
}
|
||||
}.start();
|
||||
|
||||
|
||||
new Thread() {
|
||||
public void run() {
|
||||
while (true) {
|
||||
try {
|
||||
Thread.sleep(500);
|
||||
|
||||
System.out.println("thread 2");
|
||||
|
||||
Person person = new Person("c4");
|
||||
System.out.println(person.getName());
|
||||
person.toString();
|
||||
} catch (InterruptedException e) {
|
||||
}
|
||||
}
|
||||
}
|
||||
}.start();
|
||||
|
||||
change();
|
||||
while (true) {
|
||||
try {
|
||||
Thread.sleep(1000);
|
||||
} catch (InterruptedException e) {
|
||||
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
public static void change() {
|
||||
value++;
|
||||
System.out.println(value);
|
||||
}
|
||||
|
||||
}
|
|
@ -1,14 +0,0 @@
|
|||
package com.microsoft.debug;
|
||||
|
||||
public class NameProvider {
|
||||
|
||||
public String fieldName;
|
||||
|
||||
public NameProvider(String fieldName) {
|
||||
this.fieldName = fieldName;
|
||||
}
|
||||
|
||||
public String getName() {
|
||||
return " + Provider" + this.fieldName;
|
||||
}
|
||||
}
|
|
@ -1,30 +0,0 @@
|
|||
package com.microsoft.debug;
|
||||
|
||||
public class Person {
|
||||
|
||||
private String name;
|
||||
|
||||
public Person(String name) {
|
||||
this.name = name;
|
||||
}
|
||||
|
||||
public String getName() {
|
||||
String res = "old";
|
||||
for (int i = 0; i < 2; i++) {
|
||||
res += i;
|
||||
}
|
||||
res += this.getInternalName();
|
||||
return res;
|
||||
}
|
||||
|
||||
public void setName(String name) {
|
||||
this.name = name;
|
||||
}
|
||||
|
||||
private String getInternalName() {
|
||||
int i = 2;
|
||||
NameProvider provider = new NameProvider("");
|
||||
i++;
|
||||
return provider.getName();
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>HelloWorld</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,11 +0,0 @@
|
|||
eclipse.preferences.version=1
|
||||
org.eclipse.jdt.core.compiler.codegen.inlineJsrBytecode=enabled
|
||||
org.eclipse.jdt.core.compiler.codegen.targetPlatform=1.8
|
||||
org.eclipse.jdt.core.compiler.codegen.unusedLocal=preserve
|
||||
org.eclipse.jdt.core.compiler.compliance=1.8
|
||||
org.eclipse.jdt.core.compiler.debug.lineNumber=generate
|
||||
org.eclipse.jdt.core.compiler.debug.localVariable=generate
|
||||
org.eclipse.jdt.core.compiler.debug.sourceFile=generate
|
||||
org.eclipse.jdt.core.compiler.problem.assertIdentifier=error
|
||||
org.eclipse.jdt.core.compiler.problem.enumIdentifier=error
|
||||
org.eclipse.jdt.core.compiler.source=1.8
|
|
@ -1,64 +0,0 @@
|
|||
package com.microsoft.debug;
|
||||
|
||||
|
||||
public class HelloWorld {
|
||||
static class InnerType {
|
||||
public static void print() {
|
||||
System.out.println("Inner Type.");
|
||||
}
|
||||
}
|
||||
|
||||
private static int value = 0;
|
||||
|
||||
public static void main(String[] args) throws Exception {
|
||||
new Thread() {
|
||||
public void run() {
|
||||
while (true) {
|
||||
try {
|
||||
Thread.sleep(500);
|
||||
|
||||
System.out.println("thread 1");
|
||||
|
||||
Person person = new Person("c4");
|
||||
System.out.println(person.getName());
|
||||
person.toString();
|
||||
} catch (InterruptedException e) {
|
||||
}
|
||||
}
|
||||
}
|
||||
}.start();
|
||||
|
||||
|
||||
new Thread() {
|
||||
public void run() {
|
||||
while (true) {
|
||||
try {
|
||||
Thread.sleep(500);
|
||||
|
||||
System.out.println("thread 2");
|
||||
|
||||
Person person = new Person("c4");
|
||||
System.out.println(person.getName());
|
||||
person.toString();
|
||||
} catch (InterruptedException e) {
|
||||
}
|
||||
}
|
||||
}
|
||||
}.start();
|
||||
|
||||
change();
|
||||
while (true) {
|
||||
try {
|
||||
Thread.sleep(1000);
|
||||
} catch (InterruptedException e) {
|
||||
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
public static void change() {
|
||||
value++;
|
||||
System.out.println(value);
|
||||
}
|
||||
|
||||
}
|
|
@ -1,14 +0,0 @@
|
|||
package com.microsoft.debug;
|
||||
|
||||
public class NameProvider {
|
||||
|
||||
public String fieldName;
|
||||
|
||||
public NameProvider(String fieldName) {
|
||||
this.fieldName = fieldName;
|
||||
}
|
||||
|
||||
public String getName() {
|
||||
return " + Provider" + this.fieldName;
|
||||
}
|
||||
}
|
|
@ -1,30 +0,0 @@
|
|||
package com.microsoft.debug;
|
||||
|
||||
public class Person {
|
||||
|
||||
private String name;
|
||||
|
||||
public Person(String name) {
|
||||
this.name = name;
|
||||
}
|
||||
|
||||
public String getName() {
|
||||
String res = "old";
|
||||
for (int i = 0; i < 2; i++) {
|
||||
res += i;
|
||||
}
|
||||
res += this.getInternalName();
|
||||
return res;
|
||||
}
|
||||
|
||||
public void setName(String name) {
|
||||
this.name = name;
|
||||
}
|
||||
|
||||
private String getInternalName() {
|
||||
int i = 2;
|
||||
NameProvider provider = new NameProvider("");
|
||||
i++;
|
||||
return provider.getName();
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<classpath>
|
||||
<classpathentry kind="src" path="src/main/java"/>
|
||||
<classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8/"/>
|
||||
<classpathentry kind="output" path="bin"/>
|
||||
</classpath>
|
|
@ -1 +0,0 @@
|
|||
FILE_ENV_FOR_TEST_PLAN=Successfully loaded an env from a file.
|
|
@ -1,17 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>26.environmentVariables</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
<buildCommand>
|
||||
<name>org.eclipse.jdt.core.javabuilder</name>
|
||||
<arguments>
|
||||
</arguments>
|
||||
</buildCommand>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
<nature>org.eclipse.jdt.core.javanature</nature>
|
||||
</natures>
|
||||
</projectDescription>
|
|
@ -1,24 +0,0 @@
|
|||
{
|
||||
// Use IntelliSense to learn about possible attributes.
|
||||
// Hover to view descriptions of existing attributes.
|
||||
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
|
||||
"version": "0.2.0",
|
||||
"configurations": [
|
||||
|
||||
{
|
||||
"type": "java",
|
||||
"name": "Debug (Launch)-EnvrionmentVariable<26.environmentVariables>",
|
||||
"request": "launch",
|
||||
"cwd": "${workspaceFolder}",
|
||||
"console": "internalConsole",
|
||||
"stopOnEntry": false,
|
||||
"mainClass": "EnvrionmentVariable",
|
||||
"args": "",
|
||||
"projectName": "26.environmentVariables",
|
||||
"env": {
|
||||
"CUSTOM_ENV_FOR_TEST_PLAN": "This env is for test plan."
|
||||
},
|
||||
"envFile": "${workspaceFolder}/.env"
|
||||
}
|
||||
]
|
||||
}
|
|
@ -1,10 +0,0 @@
|
|||
public class EnvrionmentVariable {
|
||||
public static void main(String[] args) {
|
||||
String customEnv = System.getenv("CUSTOM_ENV_FOR_TEST_PLAN");
|
||||
String systemPath = System.getenv("PATH");
|
||||
String envFromFile = System.getenv("FILE_ENV_FOR_TEST_PLAN");
|
||||
System.out.println(String.format("CustomEnv: %s", customEnv));
|
||||
System.out.println(String.format("SystemPath: %s", systemPath));
|
||||
System.out.println(String.format("FileEnv: %s", envFromFile));
|
||||
}
|
||||
}
|
|
@ -1,63 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
|
||||
<modelVersion>4.0.0</modelVersion>
|
||||
|
||||
<groupId>com.github.kdvolder</groupId>
|
||||
<artifactId>hello-world-service</artifactId>
|
||||
<version>0.0.1-SNAPSHOT</version>
|
||||
<packaging>jar</packaging>
|
||||
|
||||
<name>demo</name>
|
||||
<description>Demo project for Spring Boot</description>
|
||||
|
||||
<parent>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-starter-parent</artifactId>
|
||||
<version>2.0.0.RELEASE</version>
|
||||
<relativePath/> <!-- lookup parent from repository -->
|
||||
</parent>
|
||||
|
||||
<properties>
|
||||
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
|
||||
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
|
||||
<java.version>1.8</java.version>
|
||||
</properties>
|
||||
|
||||
<dependencies>
|
||||
<dependency>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-starter-actuator</artifactId>
|
||||
</dependency>
|
||||
<dependency>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-starter-web</artifactId>
|
||||
</dependency>
|
||||
<dependency>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-starter-test</artifactId>
|
||||
<scope>test</scope>
|
||||
</dependency>
|
||||
<dependency>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-devtools</artifactId>
|
||||
<scope>runtime</scope>
|
||||
</dependency>
|
||||
<dependency>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-configuration-processor</artifactId>
|
||||
<optional>true</optional>
|
||||
</dependency>
|
||||
</dependencies>
|
||||
|
||||
<build>
|
||||
<plugins>
|
||||
<plugin>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-maven-plugin</artifactId>
|
||||
</plugin>
|
||||
</plugins>
|
||||
</build>
|
||||
|
||||
|
||||
</project>
|
|
@ -1,5 +0,0 @@
|
|||
package com.github.kdvolder.helloworldservice;
|
||||
|
||||
public class Constants {
|
||||
public static final String GREETER_ID = "greeets";
|
||||
}
|
|
@ -1,52 +0,0 @@
|
|||
package com.github.kdvolder.helloworldservice;
|
||||
|
||||
import org.springframework.beans.factory.annotation.Autowired;
|
||||
import org.springframework.beans.factory.annotation.Qualifier;
|
||||
import org.springframework.boot.SpringApplication;
|
||||
import org.springframework.boot.autoconfigure.SpringBootApplication;
|
||||
import org.springframework.context.annotation.Bean;
|
||||
import org.springframework.scheduling.annotation.EnableScheduling;
|
||||
import org.springframework.web.bind.annotation.GetMapping;
|
||||
import org.springframework.web.bind.annotation.PathVariable;
|
||||
import org.springframework.web.bind.annotation.RestController;
|
||||
|
||||
|
||||
@SpringBootApplication
|
||||
@RestController
|
||||
@EnableScheduling
|
||||
public class DemoApplication {
|
||||
|
||||
public static class Bar {
|
||||
}
|
||||
|
||||
public static class Foo {
|
||||
}
|
||||
|
||||
@Autowired(required=false) void foo(@Qualifier("foobar")Foo foo) {
|
||||
System.out.println("a Foo got injected");
|
||||
}
|
||||
|
||||
@Autowired(required=false) void bar(@Qualifier("foobar")Bar bar) {
|
||||
System.out.println("a Bar got injected");
|
||||
}
|
||||
|
||||
public static void main(String[] args) {
|
||||
SpringApplication.run(DemoApplication.class, args);
|
||||
}
|
||||
|
||||
@GetMapping(value="/")
|
||||
public String mainpage() {
|
||||
return "Hello "+System.getProperty("greeting");
|
||||
}
|
||||
|
||||
|
||||
@GetMapping(value = "/hello/{name}")
|
||||
public String getMethodName(@PathVariable String name) {
|
||||
return "Hello "+name;
|
||||
}
|
||||
|
||||
|
||||
@Bean Foo foobar() {
|
||||
return new Foo();
|
||||
}
|
||||
}
|
|
@ -1,13 +0,0 @@
|
|||
package com.github.kdvolder.helloworldservice;
|
||||
|
||||
import org.springframework.stereotype.Component;
|
||||
|
||||
|
||||
@Component
|
||||
public class Greeter {
|
||||
|
||||
String greeting(String name) {
|
||||
return "Hello "+name;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,26 +0,0 @@
|
|||
package com.github.kdvolder.helloworldservice;
|
||||
|
||||
import org.springframework.boot.context.properties.ConfigurationProperties;
|
||||
import org.springframework.boot.context.properties.DeprecatedConfigurationProperty;
|
||||
|
||||
@ConfigurationProperties("my")
|
||||
public class MyProperties {
|
||||
|
||||
private String hello;
|
||||
|
||||
/**
|
||||
* @return the hello
|
||||
*/
|
||||
@DeprecatedConfigurationProperty(replacement="new.my.hello")
|
||||
public String getHello() {
|
||||
return hello;
|
||||
}
|
||||
|
||||
/**
|
||||
* @param hello the hello to set
|
||||
*/
|
||||
public void setHello(String hello) {
|
||||
this.hello = hello;
|
||||
}
|
||||
|
||||
}
|
|
@ -1 +0,0 @@
|
|||
my.hello=Yadaya
|
|
@ -1,6 +0,0 @@
|
|||
my:
|
||||
other: stuff
|
||||
hello: blah
|
||||
new:
|
||||
my:
|
||||
other: stuff
|
|
@ -1,16 +0,0 @@
|
|||
package com.github.kdvolder.helloworldservice;
|
||||
|
||||
import org.junit.Test;
|
||||
import org.junit.runner.RunWith;
|
||||
import org.springframework.boot.test.context.SpringBootTest;
|
||||
import org.springframework.test.context.junit4.SpringRunner;
|
||||
|
||||
@RunWith(SpringRunner.class)
|
||||
@SpringBootTest
|
||||
public class DemoApplicationTests {
|
||||
|
||||
@Test
|
||||
public void contextLoads() {
|
||||
}
|
||||
|
||||
}
|
|
@ -1,5 +0,0 @@
|
|||
{
|
||||
// "java.home": "C:\\Program Files\\Java\\jdk-12",
|
||||
"java.debug.settings.showLogicalStructure": true,
|
||||
"java.debug.settings.forceBuildBeforeLaunch": false
|
||||
}
|
|
@ -1,13 +0,0 @@
|
|||
public class Java12Preview {
|
||||
|
||||
public static void main(String[] args) {
|
||||
String week = "MONDAY";
|
||||
switch (week) {
|
||||
case "MONDAY" -> {
|
||||
System.out.println("This is Monday");
|
||||
}
|
||||
case "TUESDAY" -> System.out.println("This is TUESDAY");
|
||||
default -> System.out.println("Unknown day.");
|
||||
}
|
||||
}
|
||||
}
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in New Issue