![]() |
||
---|---|---|
diagrams | ||
test | ||
.editorconfig | ||
.gitattributes | ||
.gitignore | ||
LICENSE.md | ||
README.md | ||
index.js | ||
package.json |
README.md
Merkle Tree
Construct Merkle Trees and verify via proofs in JavaScript.
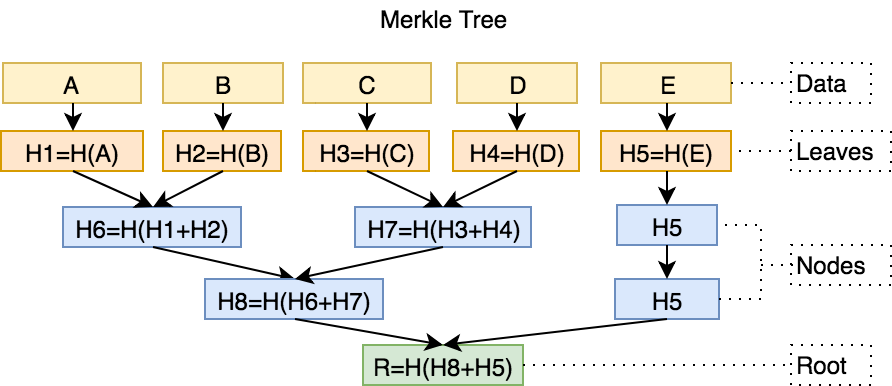
Install
npm install m-tree
Documentation
Classes
Objects
- MerkleTree :
object
Class reprensenting a Merkle Tree
MerkleTree
Kind: global class
- MerkleTree
- new MerkleTree(leaves, hashAlgo, options)
- .getLeaves() ⇒
Array
- .getLayers() ⇒
Array
- .getRoot() ⇒
Buffer
- .getProof(leaf, [index]) ⇒
Array
- .verify(proof, targetNode, root) ⇒
Boolean
new MerkleTree(leaves, hashAlgo, options)
Constructs a Merkle Tree. All nodes and leaves are stored as Buffers. Lonely leaf nodes are promoted to the next level up without being hashed again.
Param | Type | Description |
---|---|---|
leaves | Array |
Array of hashed leaves. Each leaf must be a Buffer. |
hashAlgo | function |
Algorithm used for hashing leaves and nodes |
options | Object |
Additional options |
options.isBitcoinTree | Boolean |
If set to true , generates the Merkle Tree with the Bitcoin Merkle Tree implementation. Enable it when you need to replicate Bitcoin constructed Merkle Trees. |
Example
const MerkleTree = require('m-tree')
const crypto = require('crypto')
function sha256(data) {
// returns Buffer
return crypto.createHash('sha256').update(data).digest()
}
const leaves = ['a', 'b', 'c'].map(x => sha3(x))
const tree = new MerkleTree(leaves, sha256)
merkleTree.getLeaves() ⇒ Array
Returns leaves of Merkle Tree.
Kind: instance method of MerkleTree
Returns: Array
- - array of leaves
Example
const leaves = tree.getLeaves()
merkleTree.getLayers() ⇒ Array
Returns all layers of Merkle Tree, including leaves and root.
Kind: instance method of MerkleTree
Returns: Array
- - array of layers
Example
const layers = tree.getLayers()
merkleTree.getRoot() ⇒ Buffer
Returns the Merkle root hash.
Kind: instance method of MerkleTree
Returns: Buffer
- - Merkle root hash
Example
const root = tree.getRoot()
merkleTree.getProof(leaf, [index]) ⇒ Array
Returns the proof for a target leaf.
Kind: instance method of MerkleTree
Returns: Array
- - Array of Buffer hashes.
Param | Type | Description |
---|---|---|
leaf | Buffer |
Target leaf |
[index] | Number |
Target leaf index in leaves array. Use if there are leaves containing duplicate data in order to distinguish it. |
Example
const proof = tree.getProof(leaves[2])
Example
const leaves = ['a', 'b', 'a'].map(x => sha3(x))
const tree = new MerkleTree(leaves, sha3)
const proof = tree.getProof(leaves[2], 2)
merkleTree.verify(proof, targetNode, root) ⇒ Boolean
Returns true if the proof path (array of hashes) can connect the target node to the Merkle root.
Kind: instance method of MerkleTree
Param | Type | Description |
---|---|---|
proof | Array |
Array of proof Buffer hashes that should connect target node to Merkle root. |
targetNode | Buffer |
Target node Buffer |
root | Buffer |
Merkle root Buffer |
Example
const root = tree.getRoot()
const proof = tree.getProof(leaves[2])
const verified = tree.verify(proof, leaves[2], root)
MerkleTree : object
Class reprensenting a Merkle Tree
Kind: global namespace Example
const tree = new MerkleTree(leaves, sha256)
- MerkleTree :
object
- new MerkleTree(leaves, hashAlgo, options)
- .getLeaves() ⇒
Array
- .getLayers() ⇒
Array
- .getRoot() ⇒
Buffer
- .getProof(leaf, [index]) ⇒
Array
- .verify(proof, targetNode, root) ⇒
Boolean
new MerkleTree(leaves, hashAlgo, options)
Constructs a Merkle Tree. All nodes and leaves are stored as Buffers. Lonely leaf nodes are promoted to the next level up without being hashed again.
Param | Type | Description |
---|---|---|
leaves | Array |
Array of hashed leaves. Each leaf must be a Buffer. |
hashAlgo | function |
Algorithm used for hashing leaves and nodes |
options | Object |
Additional options |
options.isBitcoinTree | Boolean |
If set to true , generates the Merkle Tree with the Bitcoin Merkle Tree implementation. Enable it when you need to replicate Bitcoin constructed Merkle Trees. |
Example
const MerkleTree = require('m-tree')
const crypto = require('crypto')
function sha256(data) {
// returns Buffer
return crypto.createHash('sha256').update(data).digest()
}
const leaves = ['a', 'b', 'c'].map(x => sha3(x))
const tree = new MerkleTree(leaves, sha256)
merkleTree.getLeaves() ⇒ Array
Returns leaves of Merkle Tree.
Kind: instance method of MerkleTree
Returns: Array
- - array of leaves
Example
const leaves = tree.getLeaves()
merkleTree.getLayers() ⇒ Array
Returns all layers of Merkle Tree, including leaves and root.
Kind: instance method of MerkleTree
Returns: Array
- - array of layers
Example
const layers = tree.getLayers()
merkleTree.getRoot() ⇒ Buffer
Returns the Merkle root hash.
Kind: instance method of MerkleTree
Returns: Buffer
- - Merkle root hash
Example
const root = tree.getRoot()
merkleTree.getProof(leaf, [index]) ⇒ Array
Returns the proof for a target leaf.
Kind: instance method of MerkleTree
Returns: Array
- - Array of Buffer hashes.
Param | Type | Description |
---|---|---|
leaf | Buffer |
Target leaf |
[index] | Number |
Target leaf index in leaves array. Use if there are leaves containing duplicate data in order to distinguish it. |
Example
const proof = tree.getProof(leaves[2])
Example
const leaves = ['a', 'b', 'a'].map(x => sha3(x))
const tree = new MerkleTree(leaves, sha3)
const proof = tree.getProof(leaves[2], 2)
merkleTree.verify(proof, targetNode, root) ⇒ Boolean
Returns true if the proof path (array of hashes) can connect the target node to the Merkle root.
Kind: instance method of MerkleTree
Param | Type | Description |
---|---|---|
proof | Array |
Array of proof Buffer hashes that should connect target node to Merkle root. |
targetNode | Buffer |
Target node Buffer |
root | Buffer |
Merkle root Buffer |
Example
const root = tree.getRoot()
const proof = tree.getProof(leaves[2])
const verified = tree.verify(proof, leaves[2], root)
Test
``bash npm test
# Credits/Resources
- [Bitcoin mining the hard way: the algorithms, protocols, and bytes](http://www.righto.com/2014/02/bitcoin-mining-hard-way-algorithms.html)
- [Raiden Merkle Tree Implemenation](https://github.com/raiden-network/raiden/blob/f9cf12571891cdf54feb4667cd2fffcb3d5daa89/raiden/mtree.py)
- [Bitcoin Talk - Merkle Trees](https://bitcointalk.org/index.php?topic=403231.msg9054025#msg9054025)
- [How Log Proofs Work](https://www.certificate-transparency.org/log-proofs-work)
- [Why aren't Solidity sha3 hashes not matching what other sha3 libraries produce?](https://ethereum.stackexchange.com/questions/559/why-arent-solidity-sha3-hashes-not-matching-what-other-sha3-libraries-produce)
# License
MIT